PyAlbum Filters
This page describes image filtering supported by PyAlbum and shows some
examples.
What is an image filter?
An image filter is a short piece of python code, which modifies an image before or after
it is resized by PyAlbum.
A filter can do anything to a picture, like placing text or logo images onto it, or modify
a picture at its whole, like blurring, sharpening or reducing color. A filter should not
change the size of the picture too much, otherwise the screen layout might be damaged.
Filters can be separately applied to thumbnail and low resolution pictures. They can be cascaded (which might lead to poor performance, if used extensively!) to combine
effects.
PyAlbum filters exist in two chains: Filter (defined by cmdline, first, album configuration)
and TFilter (defined within template.cfg). Each read in filter cascade from
one source discards the older definitions. Filters defined from the command line
have absolute precedence and disable new definitions from the other sources except
TFilters from templates.
How do I use a filter?
You can declare a filter by using the option Filter. Filters can be declared
in every option file (first, template, album).
Each filter declaration is one line with the following syntax:
Filter = XyFilter, parametername1=value1, parametername2=value2, ...
Filter = ABCFilter, parametername1=value1, parametername2=value2, ...
Each filter has a set of parameters which define its behaviour, but each filter
has some basic parameters, too, which define the behaviour of PyAlbum: when
to use a filter.
All parameter names are used in lower case.
Beware: do not use option ThumbFill=fill or crop with filters that modify the size
of the image (like BorderFilter), since filling or cropping is done after
the last filter is invoked!
To declare filters on the command line, only use single quotes inside double quotes, like this:
PyAlbum.py filter="BorderFilter, color='red,green', border='5,6', thumbnail=true"
Basic filter parameters for all filters
filtername
|
type: |
string |
default: |
Name of the filter |
|
This is just for PyAlbum's convenience and is automatically generated by
PyAlbum itself, so do not provide it as parameter! It is the name of the filter module or source file.
|
thumbnail
|
type: |
Boolean |
default: |
False |
|
Defines, if the filter is invoked on thumbnail images. Boolean values can be given
as t, true, 1, y, yes or f, false, 0, n, no.
|
lowres
|
type: |
Boolean |
default: |
False |
|
Defines, if the filter is invoked on low resolution images. Boolean values
can be given as t, true, 1, y, yes or f, false, 0, n, no. Since lowres and
thumbnail both default to False, you must at least set one to True.
|
postresize
|
type: |
Boolean |
default: |
True |
|
Defines, when the filter is invoked. "True" means, that the image
is resized first, then the filter is applied. Otherwise the order of operations
is filter first, then resize. This is seldom useful, since logos and texts
can get very small.
|
Additional filter parameters for all positioned filters
Many filters put an object onto the image, which can be text or a small image logo.
They all share the same positioning parameters.
align
|
type: |
One choice (left, center, right) |
default: |
right |
|
Defines the horizontal placement of the object.
|
valign
|
type: |
One choice (top, center, bottom) |
default: |
bottom |
|
Defines the vertical placement of the object.
|
xoffset
|
|
For left and right horizontal placement, this value defines the distance
to the image edge in pixels. This value is always positive.
|
yoffset
|
|
For top and bottom vertical placement, this value defines the distance
to the image edge in pixels. This value is always positive.
|
Provided filters
TextFilter (positioned filter)
text
|
type: | String |
default: | <empty> |
|
The text to place onto the image. If the text is too large for the image, it
is truncated.
|
color
|
type: |
Color |
default: |
black |
|
The color of the text. Colors can be given as "#rrggbb" (6 hexadecimal digits)
or "#rgb" (3 hexadecimal digits) or "r,g,b" (3 decimal numbers between 00 and 255)
or one of the 16 named W3C colors (black (#000000), silver (#C0C0C0),
gray (#808080), white (#FFFFFF), maroon (#800000),
red (#FF0000), purple (#800080), fuchsia (#FF00FF),
green (#008000), lime (#00FF00), olive (#808000),
yellow (#FFFF00), navy (#000080), blue (#0000FF),
teal (#008080) aqua (#00FFFF)).
|
font
|
type: | String |
default: | luBR12 |
|
The font for the text. This has to be the name of a PIL font without path
and extension. With option FontDir you set the path to the directory,
where your PIL fonts reside. PyAlbum has some in its "fonts" directory, but you
can download more PIL fonts from
effbot.org/downloads.
|
Filter=TextFilter, lowres=t, color=silver, align=right, valign=bottom, font=luBR18
|
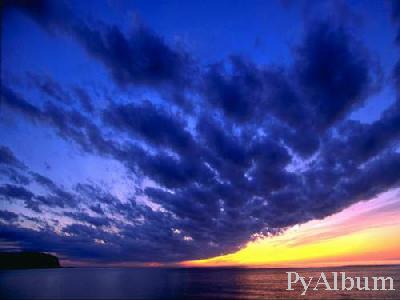 |
ShadowTextFilter (positioned filter)
text
|
type: | String |
default: | <empty> |
|
The text to place onto the image. If the text is too large for the image, it
is truncated.
|
color
|
type: |
Color |
default: |
gray |
|
The color of the text. For color values see TextFilter.
|
shadowcolor
|
type: |
Color |
default: |
black |
|
The color of the shadow text, placed shadowoffset pixels right and below the text.
For color values see TextFilter.
|
shadowoffset
|
|
The number of pixels the shadow is below and right of the text.
|
font
|
type: | String |
default: | luBR12 |
|
The font for the text. This has to be the name of a PIL font without path
and extension. On fonts see above.
|
Filter=ShadowTextFilter, lowres=t, color=#xxx, shadowcolor=black, shadowoffset=2, align=xx, valign=xx, font=luBR18
|
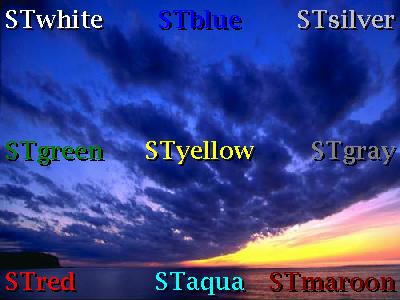 |
WatermarkFilter (positioned filter)
text
|
type: | String |
default: | <empty> |
|
The text to place onto the image. If the text is too large for the image, it
is truncated. The text is made transparent, see parameter transparency.
|
color
|
|
The color of the text. For color values see TextFilter.
|
shadowcolor
|
|
The color of the shadow text, placed shadowoffset pixels right and below the text.
For color values see TextFilter.
|
shadowoffset
|
|
The number of pixels the shadow is below and right of the text.
|
font
|
type: | String |
default: | luBR12 |
|
The font for the text. This has to be the name of a PIL font without path
and extension. On fonts see above.
|
transparency
|
type: | Integer (0 to 255) |
default: | 155 |
|
The transparency of the main text. 0 means: totally opaque, 255 means: invisible.
The shadow text is always opaque.
|
Filter=WatermarkFilter, lowres=t, color=#xxx, shadowcolor=black, shadowoffset=2, transparency=< from 25 to 225>, align =xx, valign=xx, font luBS10
|
|
Filter=WatermarkFilter, lowres=t, color "240,240,240", align=right, valign=bottom, shadowcolor=#505050, shadowoffset=1, transparency=200, font=luBS18
|
|
RotatedWatermarkFilter (positioned filter)
text
|
type: | String |
default: | <empty> |
|
The text to place onto the image. If the text is too large for the image, it
is truncated. The text is made transparent, see parameter transparency.
|
color
|
|
The color of the text. For color values see TextFilter.
|
angle
|
type: | Integer |
default: | -45 (=top left to bottom right) |
|
The angle in degrees the text is rotated. Only use align=center, valign=center
as position! Otherwise the text will be outside the image.
|
font
|
type: | String |
default: | luBS18 |
|
The font for the text. This has to be the name of a PIL font without path
and extension. On fonts see above.
|
transparency
|
type: | Integer (0 to 255) |
default: | 155 |
|
The transparency of the main text. 0 means: totally opaque, 255 means: invisible.
|
Filter=RotatedWatermarkFilter, lowres=t, text="Confidential", angle=-45, color=gray,
align=center, valign=center, transparency=100, font=luBS18
|
|
FrameFilter (standard filter)
frame
|
type: | String |
default: | <program path>/libtest/fr_StarsAndMoon.jpg |
|
An image to use as frame. It will be resized to the actual image size, so it should be
large.
|
mask
|
type: | String |
default: | <program path>/libtest/fr_StarsAndMoon_msk.gif |
|
An image to use as transparency mask. It has to be exactly the same size as the
frame image and has to be a gray scale image.
In white areas (255) of the mask, the picture will be seen, in black areas (0) the frame
is visible only. All gray values in between result a transparent area.
|
Filter=FrameFilter, lowres=t, thumbnail=f
|
|
|
|
Filter=FrameFilter, lowres=t, thumbnail=f, frame=&P/libtest/fr_Simple.jpg, mask=&P/libtest/fr_Simple_msk.gif
|
|
|
|
MaskFilter (standard filter)
mask
|
type: | String |
default: | <program path>/libtest/msk_Wall.gif |
|
An image to use as transparency mask.
In white areas (255) of the mask, the picture will be seen, in black areas (0) the
background is visible only. All gray values in between result in a mixed area.
|
bgcolor
|
|
The color, the masked out areas should be filled with. Use the same as your
web page background color and you will get nice pictures. This color
is only used, if bgtexture is not given.
|
bgtexture
|
type: | String |
default: | <empty string> |
|
Path to a texture image for the background. A new canvas with the same size as
the image to process is filled with this texture and this new canvas is used as
the background.
The texture image can be any kind of image (jpg, gif, bmp, ...) and any size.
If this parameter is is not defined, bgcolor is used as background.
|
|
Filter=MaskFilter, lowres=t, thumbnail=f, mask='&P/libtest/msk_Heart.gif', bgcolor=white
|
|
Filter=MaskFilter, lowres=t, thumbnail=f, mask='&P/libtest/msk_Wall.gif', bgcolor=white
|
|
Filter=MaskFilter, lowres=t, thumbnail=f, mask='&P/libtest/msk_Wall.gif', bgtexture='&P/libtest/Foil.bmp'
|
|
BorderFilter (standard filter)
border
|
type: | comma separated integers |
default: | <empty> |
|
A list of pixel width for the borders from inner to outer, e.g. "5" or "1,10,1".
|
color
|
type: | Color |
default: | <empty> |
|
The color(s) of the border(s). Comma separated list of color values. Count must match
count of borders, e.g. "#123456" or "gray,#770000,blue" For color values see TextFilter.
|
shadowcolor
|
|
The color of the shadow, placed shadowsize pixels right and below the image.
For color values see TextFilter.
|
shadowbgcolor
|
|
The color of the shadow background, these are 2 small triangles bottom left and
top right in the shadow area. If you set this to the background color of your
web page, a nice 3D effect can be seen. For color values see TextFilter.
|
shadowsize
|
|
The width of the shadow (bottom and right) in pixels. Leave this 0 to omit the
shadow effect and get only borders.
|
Filter=BorderFilter, lowres=t, border="10,1", color="white,black", shadowcolor=gray, shadowbgcolor=white, shadowsize=4
|
|
LogoFilter (positioned filter)
logo
|
type: | string |
default: | <programPath>/libtest/new.gif |
|
The path to a small image, which is pasted onto the image. The path may be absolute
or relative to the current directory.
You can use special tokens for defining the path. Start it with &T
to use the path to the currently used template, e.g. &T/mylogo.gif
or start it with &P to use the program path, i.e., where PyAlbum
resides. E.g. &T/libtest/PyAlbum6.gif
|
transparency
|
type: | Integer (0 to 255) |
default: | 155 |
|
The transparency of the logo image. 0 means: totally opaque, 255 means: invisible.
|
Filter=LogoFilter, lowres=t, align=xx, valign=xx, transparency=<from 0 to 210>
|
|
BWFilter (standard filter)
factor
|
|
Generate a black&white picture, reduce the color of an image. The factor has to be
between 0.0 (grayscale) and 1.0 (full color=original).
|
Filter=BWFilter, lowres=t, factor=0.0
|
|
Filter=BWFilter, factor=0.5
|
|
ContourFilter (standard filter)
Filter=ContourFilter, lowres=t
|
|
EmbossFilter (standard filter)
Filter=EmbossFilter, lowres=t
|
|
SmoothMoreFilter (standard filter)
Filter=SmoothMoreFilter, lowres=t
|
|
And there are more...
How do I code my own filter?
Only a short one here for now: take a look into the filters directory, copy the
filter that best fits your needs and give it a unique name.
The module (python program) may have any name (this is the name used in the
Filter option), the class inside has to be named PyAlbumFilter and has to inherit
from AlbumFilter.FilterBase or AlbumFilter.PositionedFilterBase.
Modify the TITLE and DESCRIPTION class attributes, and extend the ATTRS class
attribute if you need own parameters. Finally code the __init__ and doFilter methods.
Parameters are automagically available as instance attributes of the filter.
Filters must not modify the original image! Always return a modified copy.
top of page